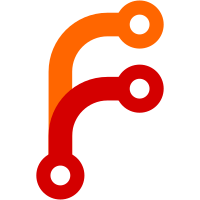
- Added support for M374 (save height map) and M375 (load height map) - Added M376 (set bed compensation taper height) - Added T parameter to G31 command - M500, M501 and M502 now use config_override.g instead of flash memory. The parameters saved and restored are: -- M307 auto tune results -- PID parameters, if you used M301 to override the auto tune PID settings -- Delta printer M665 and M666 settings -- G31 trigger height, trigger value and X and Y offsets - The M501 auto save option has been removed - Removed S and T parameters from M301 command. Use M307 command instead. - M301 with negative P parameter no longer sets bang-bang mode. Use M307 instead. - Added P parameter to the G31 command to specify Z probe type. This allows you to view the parameters for the Z probe(s) and to set parameters for a particular Z probe type without selecting that type. G31 P or G31 P0 prints the parameters of the currently-selected Z probe. - Z probe offsets are now applied during G30 probing with specified XY coordinates, including during delta auto calibration - Z probe recovery time is now applied from the end of the travel move just before probing - Fixed bad dive height when using G29 with a large trigger height - Fixed bad JSON message during printing when there were no active extruders - Added exception handlers and store a software reset code when an exception occurs - Fixed reset reason text because on the Duet WiFi a watchdog reset can look like an external reset - G30 S-1 how printes the stopped height - Implemented M401 and M402
72 lines
1.8 KiB
C++
72 lines
1.8 KiB
C++
/*
|
|
* FOPDT.h
|
|
*
|
|
* Created on: 16 Aug 2016
|
|
* Author: David
|
|
*
|
|
* Class to represent the parameters of a first order process with dead time
|
|
*/
|
|
|
|
#ifndef SRC_HEATING_FOPDT_H_
|
|
#define SRC_HEATING_FOPDT_H_
|
|
|
|
#include <cstddef>
|
|
|
|
// This is how PID parameters are stored internally
|
|
struct PidParameters
|
|
{
|
|
float kP; // controller (not model) gain
|
|
float recipTi; // reciprocal of controller integral time
|
|
float tD; // controller differential time
|
|
};
|
|
|
|
// This is how PID parameters are given in M301 commands
|
|
struct M301PidParameters
|
|
{
|
|
float kP;
|
|
float kI;
|
|
float kD;
|
|
};
|
|
|
|
class FileStore;
|
|
|
|
class FopDt
|
|
{
|
|
public:
|
|
FopDt();
|
|
|
|
bool SetParameters(float pg, float ptc, float pdt, float pMaxPwm, bool pUsePid);
|
|
|
|
float GetGain() const { return gain; }
|
|
float GetTimeConstant() const { return timeConstant; }
|
|
float GetDeadTime() const { return deadTime; }
|
|
float GetMaxPwm() const { return maxPwm; }
|
|
bool UsePid() const { return usePid; }
|
|
bool IsEnabled() const { return enabled; }
|
|
bool ArePidParametersOverridden() const { return pidParametersOverridden; }
|
|
M301PidParameters GetM301PidParameters(bool forLoadChange) const;
|
|
void SetM301PidParameters(const M301PidParameters& params);
|
|
|
|
const PidParameters& GetPidParameters(bool forLoadChange) const
|
|
{
|
|
return (forLoadChange) ? loadChangeParams : setpointChangeParams;
|
|
}
|
|
|
|
bool WriteParameters(FileStore *f, size_t heater) const; // Write the model parameters to file returning true if no error
|
|
|
|
private:
|
|
void CalcPidConstants();
|
|
|
|
float gain;
|
|
float timeConstant;
|
|
float deadTime;
|
|
float maxPwm;
|
|
bool enabled;
|
|
bool usePid;
|
|
bool pidParametersOverridden;
|
|
|
|
PidParameters setpointChangeParams; // parameters for handling changes in the setpoint
|
|
PidParameters loadChangeParams; // parameters for handling changes in the load
|
|
};
|
|
|
|
#endif /* SRC_HEATING_FOPDT_H_ */
|