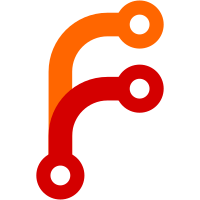
Added F (probing speed) and T (travel speed) parameters to M558 command Removed M210 command because home feed rates are defined in the homing files Increased UART interrupt priority to avoid dropping characters sent by PanelDue Bug fix: M558 P3 did not leave the Z probe control pin high Bug fix: in version 1.09j only, the move following a G92 E0 command was sometimes executed from an incorrect start point Fixed bugs with reads/writes from/to the SD card that spanned one or more whole sectors Updated to latest Atmel HSMCI driver
60 lines
1.9 KiB
C++
60 lines
1.9 KiB
C++
// This class handles input from, and output to, files.
|
|
|
|
#ifndef FILESTORE_H
|
|
#define FILESTORE_H
|
|
|
|
typedef uint32_t FilePosition;
|
|
const FilePosition noFilePosition = 0xFFFFFFFF;
|
|
const size_t FileBufLen = 256; // 512 would be more efficient, but need to free up some RAM first
|
|
|
|
class FileStore
|
|
{
|
|
public:
|
|
|
|
uint8_t Status(); // Returns OR of IOStatus
|
|
bool Read(char& b); // Read 1 byte
|
|
int Read(char* buf, unsigned int nBytes); // Read a block of nBytes length
|
|
bool Write(char b); // Write 1 byte
|
|
bool Write(const char *s, unsigned int len); // Write a block of len bytes
|
|
bool Write(const char* s); // Write a string
|
|
bool Close(); // Shut the file and tidy up
|
|
bool Seek(FilePosition pos); // Jump to pos in the file
|
|
FilePosition GetPosition() const; // Return the current position in the file, assuming we are reading the file
|
|
#if 0 // not currently used
|
|
bool GoToEnd(); // Position the file at the end (so you can write on the end).
|
|
#endif
|
|
FilePosition Length() const; // File size in bytes
|
|
float FractionRead() const; // How far in we are
|
|
void Duplicate(); // Create a second reference to this file
|
|
bool Flush(); // Write remaining buffer data
|
|
static float GetAndClearLongestWriteTime(); // Return the longest time it took to write a block to a file, in milliseconds
|
|
|
|
friend class Platform;
|
|
|
|
protected:
|
|
|
|
FileStore(Platform* p);
|
|
void Init();
|
|
bool Open(const char* directory, const char* fileName, bool write);
|
|
|
|
private:
|
|
bool ReadBuffer();
|
|
bool WriteBuffer();
|
|
bool InternalWriteBlock(const char *s, unsigned int len);
|
|
byte *GetBuffer() { return reinterpret_cast<byte*>(buf32); }
|
|
|
|
uint32_t buf32[FileBufLen/4];
|
|
Platform* platform;
|
|
unsigned int bufferPointer;
|
|
|
|
FIL file;
|
|
unsigned int lastBufferEntry;
|
|
unsigned int openCount;
|
|
|
|
bool inUse;
|
|
bool writing;
|
|
|
|
static uint32_t longestWriteTime;
|
|
};
|
|
|
|
#endif
|