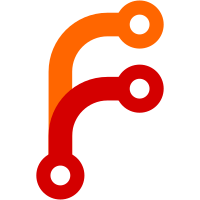
Implemented F, H and R parameters to M106 command. The second fan output on a Duet 0.8.5 now defaults to being a thermostatic fan at power up. Improved speed of file upload to SD card G32 is now allowed if the printer has not been homed, if there is a bed.g file G30 commands are no longer allowed on a delta that has not been homed M572 parameter P (drive number) replaced by parameter D (extruder number) File info requests are now processed in stages to reduce impact on printing (thanks chrishamm) Use latest network stack and webserver modules from chrishamm (thanks chrishamm) Added Roland mill support (thanks RRP/chrishamm) Added S parameter (idle timeout) to M18 ans M84 commands (thanks chrishamm) Moved I/O pin assignments to separate Pins.h file to more easily support alternative hardware (thanks dnewman) Bug fix: filament usage and % print complete figures were incorrect when absolute extruder coordinates were used Bug fix: file-based print estimate was occasionally returned as 'inf' which caused the web interface to disconnect Bug fix: M666 now flags all towers as not homed Bug fixes to extruder pressure compensation (M572 command).
69 lines
2 KiB
C++
69 lines
2 KiB
C++
// This class handles input from, and output to, files.
|
|
|
|
#ifndef FILESTORE_H
|
|
#define FILESTORE_H
|
|
|
|
typedef uint32_t FilePosition;
|
|
const FilePosition noFilePosition = 0xFFFFFFFF;
|
|
const size_t FileBufLen = 256; // 512 would be more efficient, but need to free up some RAM first
|
|
|
|
enum class IOStatus : uint8_t
|
|
{
|
|
nothing = 0,
|
|
byteAvailable = 1,
|
|
atEoF = 2,
|
|
clientLive = 4,
|
|
clientConnected = 8
|
|
};
|
|
|
|
class FileStore
|
|
{
|
|
public:
|
|
|
|
uint8_t Status(); // Returns OR of IOStatus
|
|
bool Read(char& b); // Read 1 byte
|
|
int Read(char* buf, unsigned int nBytes); // Read a block of nBytes length
|
|
bool Write(char b); // Write 1 byte
|
|
bool Write(const char *s, unsigned int len); // Write a block of len bytes
|
|
bool Write(const char* s); // Write a string
|
|
bool Close(); // Shut the file and tidy up
|
|
bool Seek(FilePosition pos); // Jump to pos in the file
|
|
FilePosition Position() const; // Return the current position in the file, assuming we are reading the file
|
|
#if 0 // not currently used
|
|
bool GoToEnd(); // Position the file at the end (so you can write on the end).
|
|
#endif
|
|
FilePosition Length() const; // File size in bytes
|
|
float FractionRead() const; // How far in we are
|
|
void Duplicate(); // Create a second reference to this file
|
|
bool Flush(); // Write remaining buffer data
|
|
static float GetAndClearLongestWriteTime(); // Return the longest time it took to write a block to a file, in milliseconds
|
|
|
|
friend class Platform;
|
|
|
|
protected:
|
|
|
|
FileStore(Platform* p);
|
|
void Init();
|
|
bool Open(const char* directory, const char* fileName, bool write);
|
|
|
|
private:
|
|
bool ReadBuffer();
|
|
bool WriteBuffer();
|
|
bool InternalWriteBlock(const char *s, unsigned int len);
|
|
byte *GetBuffer() { return reinterpret_cast<byte*>(buf32); }
|
|
|
|
uint32_t buf32[FileBufLen/4];
|
|
Platform* platform;
|
|
unsigned int bufferPointer;
|
|
|
|
FIL file;
|
|
unsigned int lastBufferEntry;
|
|
unsigned int openCount;
|
|
|
|
bool inUse;
|
|
bool writing;
|
|
|
|
static uint32_t longestWriteTime;
|
|
};
|
|
|
|
#endif
|