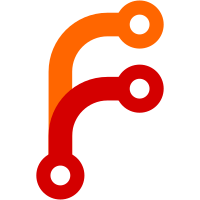
New features ============ The PWM frequency for the heated bed and for any heater used as a chamber heater is now 10Hz for bettercompatibility with DC-AC SSRs. The PWM frequency for fans is now configurable using the F paramete ron the M106 command. The default is 500Hz, which gives esonable control of fans not designed for PWM. Increase it to 25000Hz when using 4-wire PWM fans. When a Duet 0.8.5 board is configured or detected, the fan control is now automatically inverted. If you previously used M106 P0 I1 in config.g to invert it, you will need to remove that. M579 (scale Cartesian axes) is now implemented (thanks chrishamm). M114, M119 and M573 commands can now be executed concurrently with other commands. When DDA debugging is enabled, the debug output now includes all active extruders instead of just the first two. M408 S0 now includes the fan speeds (for PanelDue). M119 now reports the Z probe as well as the endstop switch states. A tool can now be defined even if a tool with the same tool number exists already. The existing tool will be shut down and deleted. The bed heater can now be disabled using M140 S-1 (thanks chrishamm). The chamber heater (if present) and the endstop switch states are now reported to the web interface (thanks chrishamm). Increased defauklt Z prove dive height to 5mm. Increased default PID Ki to 0.2 Bug fixes ========= On a CoreXY machine, XY speeds were too low by a factor of sqrt(2). On a delta machine, after running auto calibration the Z=0 height could be slightly inaccurate, depending on the difference between the X and Z endstop corrections When using a non-intelligent modulated Z probe on a Duet 0.8.5, the modulation pin number was incorrect. The M27 (Report SD card print status) response was inverted compared to what it should be. When in Marlin mode it now includes the "byte n/m" field that some versions of Pronterface expect. Cold extrusion prevention did not work - an error message was generated, but the extruder was driven anyway. M999 PERASE is now more reliable (thanks chrishamm). M23, M30 and M32 commands did not work when the filename parameter passed included an absolute path. //A T command inside a macro file did not execute the tool change macros files. A memory leak occurred when a tool was deleted. All moves are now completed before switching to CoreXY mode. Polling requests from PanelDue were not relied to when a macro was being executed M667 with no parameters returned an incorrect string
335 lines
7.4 KiB
C++
335 lines
7.4 KiB
C++
/****************************************************************************************************
|
|
|
|
RepRapFirmware - Tool
|
|
|
|
This class implements a tool in the RepRap machine, usually (though not necessarily) an extruder.
|
|
|
|
Tools may have zero or more drives associated with them and zero or more heaters. There are a fixed number
|
|
of tools in a given RepRap, with fixed heaters and drives. All this is specified on reboot, and cannot
|
|
be altered dynamically. This restriction may be lifted in the future. Tool descriptions are stored in
|
|
GCode macros that are loaded on reboot.
|
|
|
|
-----------------------------------------------------------------------------------------------------
|
|
|
|
Version 0.1
|
|
|
|
Created on: Apr 11, 2014
|
|
|
|
Adrian Bowyer
|
|
RepRap Professional Ltd
|
|
http://reprappro.com
|
|
|
|
Licence: GPL
|
|
|
|
****************************************************************************************************/
|
|
|
|
#include "RepRapFirmware.h"
|
|
|
|
Tool * Tool::freelist = nullptr;
|
|
|
|
/*static*/ Tool * Tool::Create(int toolNumber, long d[], size_t dCount, long h[], size_t hCount)
|
|
{
|
|
if (dCount > DRIVES - AXES)
|
|
{
|
|
reprap.GetPlatform()->Message(BOTH_ERROR_MESSAGE, "Tool creation: attempt to use more drives than there are in the RepRap...");
|
|
return nullptr;
|
|
}
|
|
|
|
if (hCount > HEATERS)
|
|
{
|
|
reprap.GetPlatform()->Message(BOTH_ERROR_MESSAGE, "Tool creation: attempt to use more heaters than there are in the RepRap...");
|
|
return nullptr;
|
|
}
|
|
|
|
Tool *t;
|
|
if (freelist != nullptr)
|
|
{
|
|
t = freelist;
|
|
freelist = t->next;
|
|
}
|
|
else
|
|
{
|
|
t = new Tool;
|
|
}
|
|
|
|
t->myNumber = toolNumber;
|
|
t->next = nullptr;
|
|
t->active = false;
|
|
t->driveCount = dCount;
|
|
t->heaterCount = hCount;
|
|
t->heaterFault = false;
|
|
t->mixing = false;
|
|
t->displayColdExtrudeWarning = false;
|
|
|
|
for(size_t axis = 0; axis < AXES; axis++)
|
|
{
|
|
t->offset[axis] = 0.0;
|
|
}
|
|
|
|
if (t->driveCount > 0)
|
|
{
|
|
float r = 1.0/(float)(t->driveCount);
|
|
|
|
for (size_t drive = 0; drive < t->driveCount; drive++)
|
|
{
|
|
t->drives[drive] = d[drive];
|
|
t->mix[drive] = r;
|
|
}
|
|
}
|
|
|
|
if (t->heaterCount > 0)
|
|
{
|
|
for(size_t heater = 0; heater < t->heaterCount; heater++)
|
|
{
|
|
t->heaters[heater] = h[heater];
|
|
t->activeTemperatures[heater] = ABS_ZERO;
|
|
t->standbyTemperatures[heater] = ABS_ZERO;
|
|
}
|
|
}
|
|
|
|
return t;
|
|
}
|
|
|
|
/*static*/ void Tool::Delete(Tool *t)
|
|
{
|
|
if (t != nullptr)
|
|
{
|
|
t->next = freelist;
|
|
freelist = t;
|
|
}
|
|
}
|
|
|
|
void Tool::Print(StringRef& reply)
|
|
{
|
|
reply.printf("Tool %d - drives: ", myNumber);
|
|
char comma = ',';
|
|
for (size_t drive = 0; drive < driveCount; drive++)
|
|
{
|
|
if (drive >= driveCount - 1)
|
|
{
|
|
comma = ';';
|
|
}
|
|
reply.catf("%d%c", drives[drive], comma);
|
|
}
|
|
|
|
reply.cat(" heaters (active/standby temps): ");
|
|
comma = ',';
|
|
for (size_t heater = 0; heater < heaterCount; heater++)
|
|
{
|
|
if (heater >= heaterCount - 1)
|
|
{
|
|
comma = ';';
|
|
}
|
|
reply.catf("%d (%.1f/%.1f)%c", heaters[heater],
|
|
activeTemperatures[heater], standbyTemperatures[heater], comma);
|
|
}
|
|
|
|
reply.catf(" status: %s", active ? "selected" : "standby");
|
|
}
|
|
|
|
float Tool::MaxFeedrate() const
|
|
{
|
|
if (driveCount <= 0)
|
|
{
|
|
reprap.GetPlatform()->Message(BOTH_ERROR_MESSAGE, "Attempt to get maximum feedrate for a tool with no drives.\n");
|
|
return 1.0;
|
|
}
|
|
float result = 0.0;
|
|
for (size_t d = 0; d < driveCount; d++)
|
|
{
|
|
float mf = reprap.GetPlatform()->MaxFeedrate(drives[d] + AXES);
|
|
if (mf > result)
|
|
{
|
|
result = mf;
|
|
}
|
|
}
|
|
return result;
|
|
}
|
|
|
|
float Tool::InstantDv() const
|
|
{
|
|
if (driveCount <= 0)
|
|
{
|
|
reprap.GetPlatform()->Message(BOTH_ERROR_MESSAGE, "Attempt to get InstantDv for a tool with no drives.\n");
|
|
return 1.0;
|
|
}
|
|
float result = FLT_MAX;
|
|
for (size_t d = 0; d < driveCount; d++)
|
|
{
|
|
float idv = reprap.GetPlatform()->ActualInstantDv(drives[d] + AXES);
|
|
if (idv < result)
|
|
{
|
|
result = idv;
|
|
}
|
|
}
|
|
return result;
|
|
}
|
|
|
|
// There is a temperature fault on a heater.
|
|
// Disable all tools using that heater.
|
|
// This function must be called for the first
|
|
// entry in the linked list.
|
|
|
|
void Tool::FlagTemperatureFault(int8_t heater)
|
|
{
|
|
Tool* n = this;
|
|
while(n != nullptr)
|
|
{
|
|
n->SetTemperatureFault(heater);
|
|
n = n->Next();
|
|
}
|
|
}
|
|
|
|
void Tool::ClearTemperatureFault(int8_t heater)
|
|
{
|
|
Tool* n = this;
|
|
while(n != nullptr)
|
|
{
|
|
n->ResetTemperatureFault(heater);
|
|
n = n->Next();
|
|
}
|
|
}
|
|
|
|
void Tool::SetTemperatureFault(int8_t dudHeater)
|
|
{
|
|
for (size_t heater = 0; heater < heaterCount; heater++)
|
|
{
|
|
if(dudHeater == heaters[heater])
|
|
{
|
|
heaterFault = true;
|
|
return;
|
|
}
|
|
}
|
|
}
|
|
|
|
void Tool::ResetTemperatureFault(int8_t wasDudHeater)
|
|
{
|
|
for (size_t heater = 0; heater < heaterCount; heater++)
|
|
{
|
|
if (wasDudHeater == heaters[heater])
|
|
{
|
|
heaterFault = false;
|
|
return;
|
|
}
|
|
}
|
|
}
|
|
|
|
bool Tool::AllHeatersAtHighTemperature(bool forExtrusion) const
|
|
{
|
|
for (size_t heater = 0; heater < heaterCount; heater++)
|
|
{
|
|
const float temperature = reprap.GetHeat()->GetTemperature(heaters[heater]);
|
|
if (temperature < HOT_ENOUGH_TO_RETRACT || (temperature < HOT_ENOUGH_TO_EXTRUDE && forExtrusion))
|
|
{
|
|
return false;
|
|
}
|
|
}
|
|
return true;
|
|
}
|
|
|
|
void Tool::Activate(Tool* currentlyActive)
|
|
{
|
|
if (!active)
|
|
{
|
|
if (currentlyActive != nullptr && currentlyActive != this)
|
|
{
|
|
currentlyActive->Standby();
|
|
}
|
|
for (size_t heater = 0; heater < heaterCount; heater++)
|
|
{
|
|
reprap.GetHeat()->SetActiveTemperature(heaters[heater], activeTemperatures[heater]);
|
|
reprap.GetHeat()->SetStandbyTemperature(heaters[heater], standbyTemperatures[heater]);
|
|
reprap.GetHeat()->Activate(heaters[heater]);
|
|
}
|
|
active = true;
|
|
}
|
|
}
|
|
|
|
void Tool::Standby()
|
|
{
|
|
if (active)
|
|
{
|
|
for (size_t heater = 0; heater < heaterCount; heater++)
|
|
{
|
|
reprap.GetHeat()->SetStandbyTemperature(heaters[heater], standbyTemperatures[heater]);
|
|
reprap.GetHeat()->Standby(heaters[heater]);
|
|
}
|
|
active = false;
|
|
}
|
|
}
|
|
|
|
void Tool::SetVariables(const float* standby, const float* active)
|
|
{
|
|
for (size_t heater = 0; heater < heaterCount; heater++)
|
|
{
|
|
if (active[heater] < NEARLY_ABS_ZERO && standby[heater] < NEARLY_ABS_ZERO)
|
|
{
|
|
// Temperatures close to ABS_ZERO turn off all associated heaters
|
|
reprap.GetHeat()->SwitchOff(heaters[heater]);
|
|
}
|
|
else
|
|
{
|
|
if (active[heater] < BAD_HIGH_TEMPERATURE)
|
|
{
|
|
activeTemperatures[heater] = active[heater];
|
|
reprap.GetHeat()->SetActiveTemperature(heaters[heater], activeTemperatures[heater]);
|
|
}
|
|
if (standby[heater] < BAD_HIGH_TEMPERATURE)
|
|
{
|
|
standbyTemperatures[heater] = standby[heater];
|
|
reprap.GetHeat()->SetStandbyTemperature(heaters[heater], standbyTemperatures[heater]);
|
|
}
|
|
}
|
|
}
|
|
}
|
|
|
|
void Tool::GetVariables(float* standby, float* active) const
|
|
{
|
|
for (size_t heater = 0; heater < heaterCount; heater++)
|
|
{
|
|
active[heater] = activeTemperatures[heater];
|
|
standby[heater] = standbyTemperatures[heater];
|
|
}
|
|
}
|
|
|
|
// May be called from ISR
|
|
bool Tool::ToolCanDrive(bool extrude)
|
|
{
|
|
if (heaterFault)
|
|
return false;
|
|
|
|
if (reprap.ColdExtrude() || AllHeatersAtHighTemperature(extrude))
|
|
return true;
|
|
|
|
displayColdExtrudeWarning = true;
|
|
return false;
|
|
}
|
|
|
|
// Update the number of active drives and extruders in use to reflect what this tool uses
|
|
void Tool::UpdateExtruderAndHeaterCount(uint16_t &numExtruders, uint16_t &numHeaters) const
|
|
{
|
|
for (size_t drive = 0; drive < driveCount; drive++)
|
|
{
|
|
if (drives[drive] >= numExtruders)
|
|
{
|
|
numExtruders = drives[drive] + 1;
|
|
}
|
|
}
|
|
|
|
for (size_t heater = 0; heater < heaterCount; heater++)
|
|
{
|
|
if (heaters[heater] != BED_HEATER && heaters[heater] >= numHeaters)
|
|
{
|
|
numHeaters = heaters[heater] + 1;
|
|
}
|
|
}
|
|
}
|
|
|
|
bool Tool::DisplayColdExtrudeWarning()
|
|
{
|
|
bool result = displayColdExtrudeWarning;
|
|
displayColdExtrudeWarning = false;
|
|
return result;
|
|
}
|
|
|
|
// End
|